Featured Contents
Latest Posts
Fibonacci Java Program
Java Program: Simple Multiplication Table
Simple Addition, Division, and Do While in Java
Char Sorting: Ascending and Descending in Java
import java.io.*;
import java.util.*;
public class charSorting
{
private static PrintStream pp=System.out;
public static BufferedReader br=new BufferedReader(new InputStreamReader(System.in));
public static void main(String args[])throws IOException
{
char[] a=new char[5];
int x,y;
for (x=0;x
{
pp.println("You Entered a String!");
x=x-1;continue;
}
else
a[x]=b.charAt(0);
}
pp.println();
pp.print("Given Letter:");
for (y=0;y
pp.print(a[y]+" ");
}
}
Multiples of a Number

Reversed Numbers in Java
package javaapplication1;
public class ReservedNumbers {
public static void main(String[] args) {
int n = 12345;
int t,r = 0;
System.out.println("The original numbers : " + n);
do
{
t = n % 10;
r = r * 10 + t;
n = n / 10;
}
while (n > 0);
System.out.println("The reversed numbers : " + r);
}
}
How to Open Edit Window for Programming
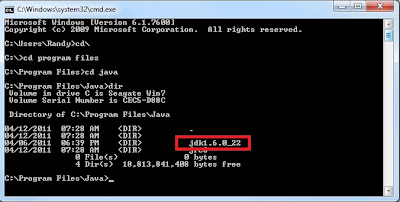
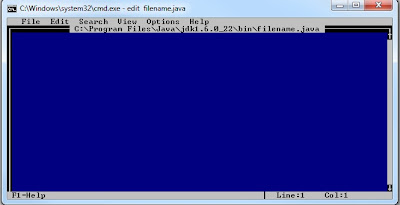
Java Program: X and Y axis


package javaapplication1; import java.io.*; public class N8 { public static BufferedReader q=new BufferedReader (new InputStreamReader (System.in)); public static void main (String []args)throws IOException{ System.out.print("Input x: "); String X=q.readLine(); int x=Integer.parseInt(X); System.out.print("Input y: "); String Y=q.readLine(); int y=Integer.parseInt(Y); if (x>0 && y>0) { System.out.print("Quadrant 1"); } else if (x<0 && y>0) { System.out.print("Quadrant 2"); } else if (x<0 && y<0) { System.out.print("Quadrant 3"); } else if (x>0 && y<0) { System.out.print("Quadrant 4"); } else if (x==0 && y<0) { System.out.print("Y axis"); } else if (x==0 && y<0 && y>0) { System.out.print("Y axis"); } else if (x<0 && y==0) { System.out.print("X axis"); } else if (x>0 && y==0) { System.out.print("X axis"); } else { System.out.print("Origin"); } } } |
Simple Calculator using Buffered Reader


import java.io.*; public class n6 { public static BufferedReader q=new BufferedReader (new InputStreamReader (System.in)); public static void main (String []args)throws IOException{ System.out.print("Enter 1st number: "); String num1=q.readLine(); int n1=Integer.parseInt(num1); System.out.print("Enter operation: "); String op=q.readLine(); char o=op.charAt(0); System.out.print("Enter 2nd number: "); String num2=q.readLine(); int n2=Integer.parseInt(num2); if (n2==0 && o=='/') { System.out.println("Error"); } else if(o == '+') { int sum=0; sum=n1+n2; System.out.println("The sum is " +sum); } else if(o == '-') { int diff=0; diff=n1-n2; System.out.println("The difference is " +diff); } else if(o == '*') { int prod=0; prod=n1*n2; System.out.println("The product is " +prod); } else if(o == '/') { double quo=0; quo=n1/n2; System.out.println("The quotient is " +quo); } else { System.out.println("Invalid"); } } } |
Numbers to words in Java
Write a program that accepts a number and output its equivalent in words.
Sample input/output:
Codes:
package javaapplication1;
import java.io.*;
public class num1 {
public static int charAt(int num, int index) {
return String.valueOf(num).charAt(index); }
private static PrintStream sys = System.out;
public static BufferedReader q = new BufferedReader (new InputStreamReader(System.in));
public static void main(String[] args) throws IOException {
String[] units = {null, "one", "two", "three", "four", "five", "six", "seven", "eight", "nine"};
String[] tenths = {"ten", "eleven", "twelve", "thirteen", "fourteen", "fifteen", "sixteen",
"seventeen", "eighteen", "nineteen"};
String[] special = {null, null, "twenty", "thirty", "fourty", "fifty", "sixty", "seventy", "eighty", "ninety"};
String[] hundreds = {null, "one hundred", "two hundred", "three hundred", "four hundred", "five hundred",
"six hundred ", "seven hundred", "eight hundred", "nine hundred"};
String[] thousands = {null, "one thousand", "two thousand", "three thousand", "four thousand",
"five thousand","six thousand", "seven thousand", "eight thousand", "nine thousand"};
int y = 0, num = 0;
do {
sys.print("Enter a number: "); num = Integer.parseInt(q.readLine());
if (num < 10000)
y = 1;
} while (y==0);
int x = Integer.toString(num).length();
switch (x)
{
case 1: {
if (num == 0)
sys.println("zero");
else
sys.println(units[num]);
break; }
case 2: {
if (charAt(num,0) - '0' == 1)
sys.println(tenths[charAt(num,1) - '0']);
else
{
sys.print(special[charAt(num,0) - '0']+" ");
sys.println(units[charAt(num,1) - '0']);
}
break; }
case 3: {
sys.print(hundreds[charAt(num,0) - '0']+" ");
{
if (charAt(num,1) - '0' == 1)
sys.print(tenths[charAt(num, 2) - '0']);
else if (charAt(num,1) - '0' == 0)
{ if (charAt(num, 2) - '0' != 0)
sys.print(units[charAt(num, 2) - '0']); }
else
{
sys.print(special[charAt(num, 1) - '0'] + " ");
if (charAt(num,2) - '0' != 0)
sys.print(units[charAt(num,2) - '0']);
}
}
sys.println();
break; }
case 4: {
sys.print(thousands[charAt(num,0) - '0']+" ");
if (charAt(num,1) - '0' != 0)
sys.print(hundreds[charAt(num,1) - '0']+" ");
{
if (charAt(num,2) - '0' == 1)
sys.print(tenths[charAt(num, 3) - '0']);
else if (charAt(num,2) - '0' == 0)
{ if (charAt(num, 3) - '0' != 0)
sys.print(units[charAt(num, 3) - '0']); }
else
{
sys.print(special[charAt(num, 2) - '0'] + " ");
if (charAt(num,3) - '0' != 0)
sys.print(units[charAt(num,3) - '0']);
}
}
sys.println();
break; }
}
}
}
Popular Posts
-
Problem: Write a program that mimics a calculator. The program should take as input two integers and the operation to be performed. It shoul...
-
Problem: Write a program that calculates and prints the bill for a cellular telephone company. The company offers two types of service: regu...
-
Problem: Write a program that prompts the user to input the x-y coordinate of a point in a Cartesian plane. The program should then output a...
-
Machine Problem: Create a Multiplication Table that ranges from 2 to 12 only. Output: Source Code: import java.io.*; public class Mu...
-
Create a java program that will sort the characters in ascending and descending order Output: Source Code: import java.io.*; import java.uti...
-
Buffered Reader Problem: Write a program that accepts a number and output its equivalent in words. Sample input/output: Codes: package jav...
-
Create a Program that will add or divide, then ask the user if they'd want to perform the computation again using Do While Output:...
-
Output: Source Code: import java.io.*; public class Fibonacci { private static PrintStream p=System.out; public stati...
-
How to open an edit Window: Note: Make sure you have a Java bin . Go to command prompt and encode the following: 1. cd\ 2. cd Program Files...
-
Problem: Write a program that will output the multiples of a number. Arrange it from lowest to highest. Sample Output: Source Codes: package...